jQuery Plugins
Useful light jQuery plugins that are handy to get the job done.
Method |
---|
$.fn.firstItem() |
Get first item in list or null if no items in list. |
$.fn.lastItem() |
Get last item in list or null if no items in list. |
$.fn.isChecked() |
Determines if any object is a checkbox that is checked. |
$.fn.setChecked( bValue ) |
Set checked status of all checkboxes. |
$.fn.tableSort( oOptions ) |
Identify and define headers in a table for sorting. |
$.fn.layoutBox( oOptions ) |
Get current computed box metrics for the first element in the set of matched elements. |
$.fn.sizeControl( oOptions ) |
Controls height, width or both of an element to fill a container. |
$.fn.emptyTextInput( oOptions ) |
Manages empty message for a text input field. |
$.fn.setFocus( oOptions ) |
Set focus asynchronously on the first element specified until object is defined and actually get focus or the specified amount of time has elapsed. |
$.fn.tocControl( oOptions ) $.fn.tocControl( sCommand [, oOptions] ) |
Manage a UL list with child UL lists as a TOC tree structure. |
function $.fn.firstItem()
Get first item in list or null if no items in list.
- (return)
- First item in list or null if no items in list.
This method is useful for indexing the first element in a jQuery list without testing for length. The following is equivalent.
// Normal method to get first element. var oElement = null; var jqList = $("div.something"); if (jqList.length > 0) oElement = jqList[0]; // Use this method instead. var oElement = $("div.something").firstItem();
function $.fn.lastItem()
Get last item in list or null if no items in list.
- (return)
- Last item in list or null if no items in list.
This method is useful for indexing the last element in a jQuery list without testing for length. The following is equivalent.
// Normal method to get first element. var oElement = null; var jqList = $("div.something"); if (jqList.length > 0) oElement = jqList[jqList.length-1]; // Use this method instead. var oElement = $("div.something").lastItem();
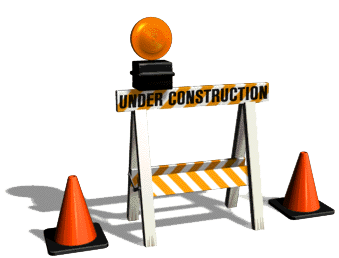